For all of my courses I include the Three.JS library I used at the time I was writing and recording the course. This ensures the code matches the library so no further installation is required other than downloading and unzipping a zip file from Udemy or GitHub or cloning and forking a repo from GitHub. But another approach is to use a package manager. By far the most popular is NPM, Node Package Manager and in this article we’ll look at using this approach.
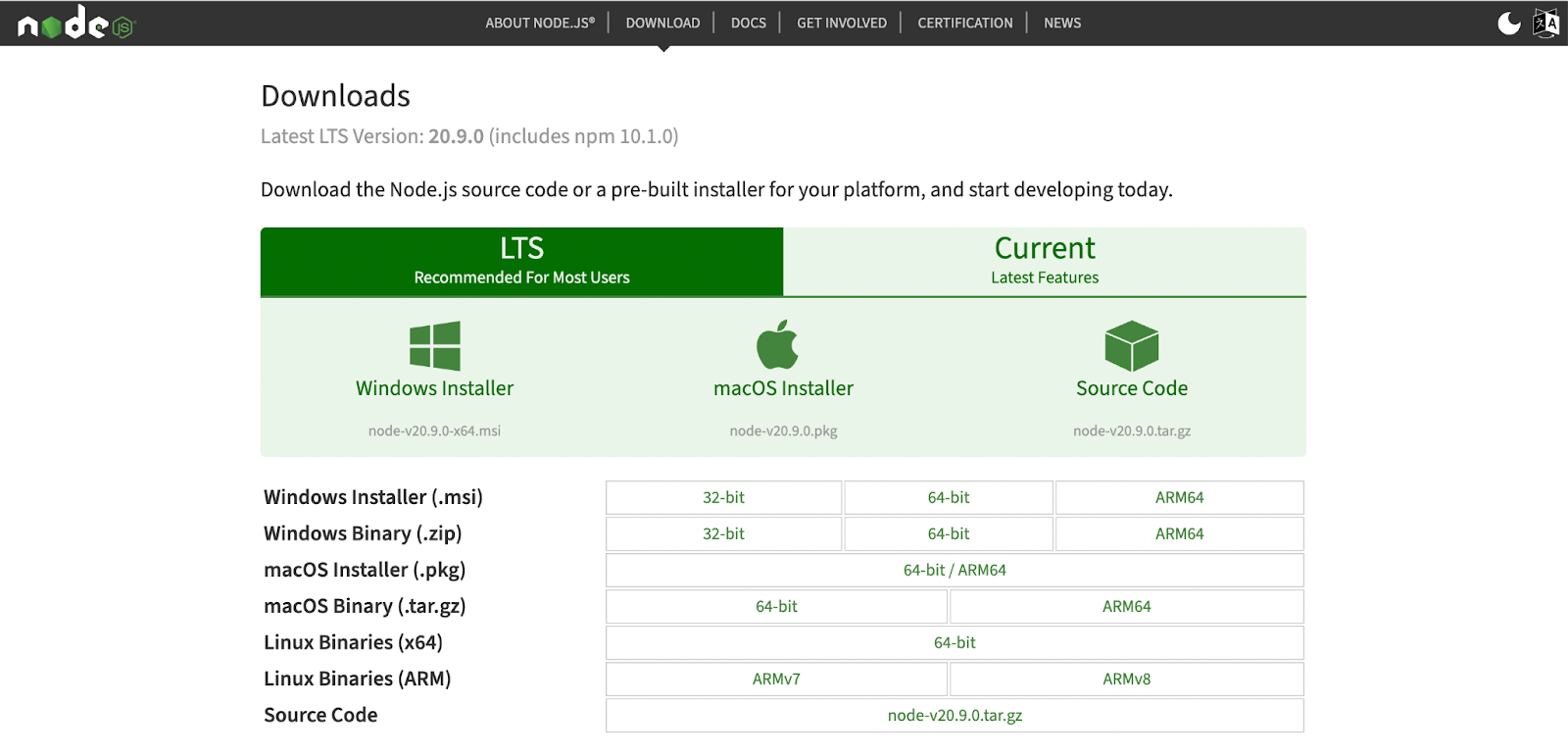
To start you will need Node.JS installed on your PC, Mac or Linux device. If you haven’t got Node.JS installed then click the Node.JS link above or enter nodejs.org in your browser address bar. Download the installer for your device and install. NPM comes with the install.
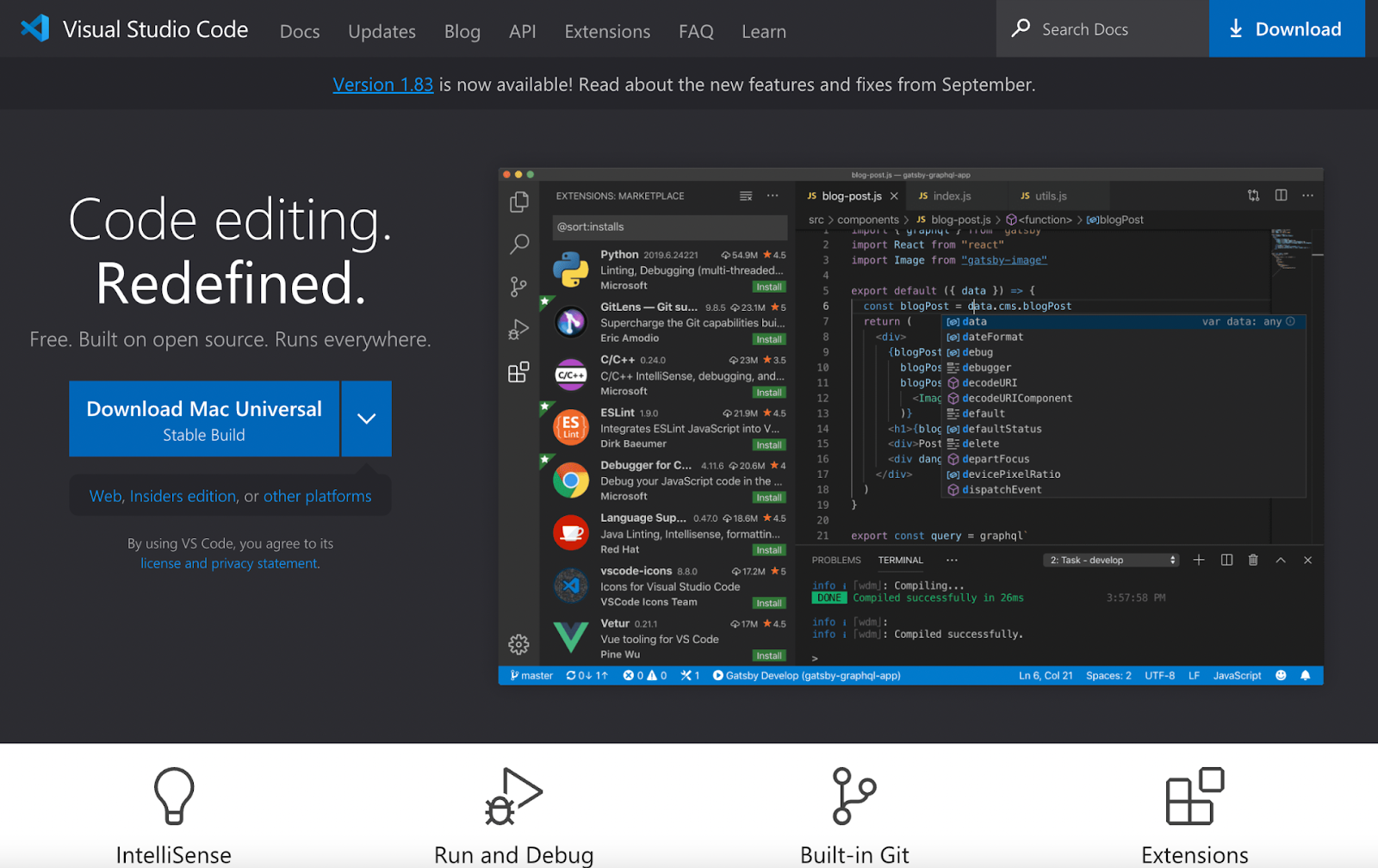
If you haven’t got VSCode installed then install that as well. It is my recommended code editor these days. Either click the link above or enter https://code.visualstudio.com/ in your browser address bar.
Open VSCode and choose Open.
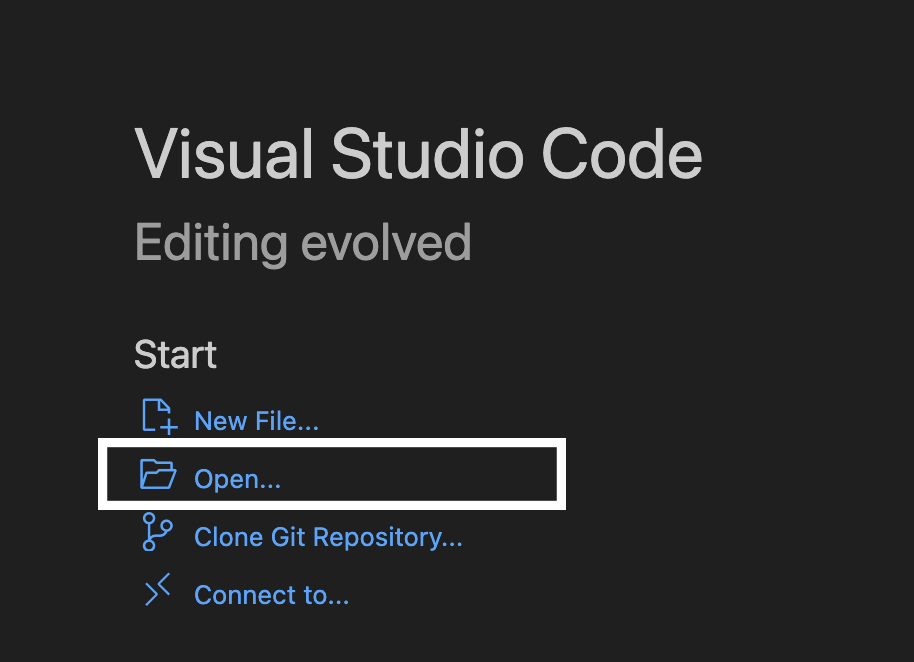
Navigate to a new folder where your project files will be stored. You’ll need to agree to trust the authors, but since that is you there is no problem. Use menu: Terminal > New Terminal. Enter
npm install three
Notice you now have a node_modules folder and two new files package.json and package-lock.json.
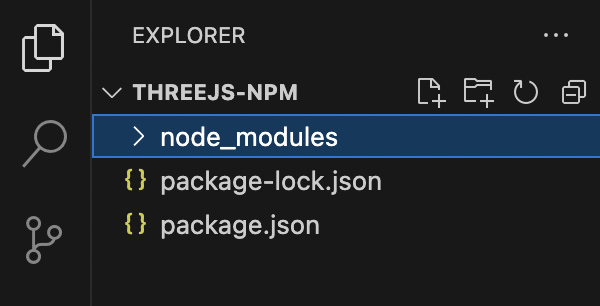
package.json looks like this. three is listed as a dependency. package-lock.json is created and edited by npm and should not be touched.
{
"dependencies": {
"three": "^0.157.0"
}
}
three is the Three.JS library which you’ll find in the node_modules/three folder.
Now we’re going to add the build tool vite. Enter
npm install -D vite
Several new folders are added to the node_modules folder including one called vite. The others are dependencies that vite relies on.
Open package.json and add
"type": "module",
"scripts": {
"dev": "vite",
"build": "vite build"
},
This will allow you to launch a dev server and package a completed project for distribution.
You could place your project files at the root of the folder. But most developers prefer to keep things tidy by adding content to folders. Create a src folder and a public folder and create a new file called vite.config.js add this code to the file.
export default {
root: "src",
publicDir: "../public",
build: {
outDir: "../build"
}
};
Now vite will look in src for any html or js files, in public for assets and package for distribution to the build folder. Note the public and build paths are relative to the src path.
To see an example using npm and vite download this repo.
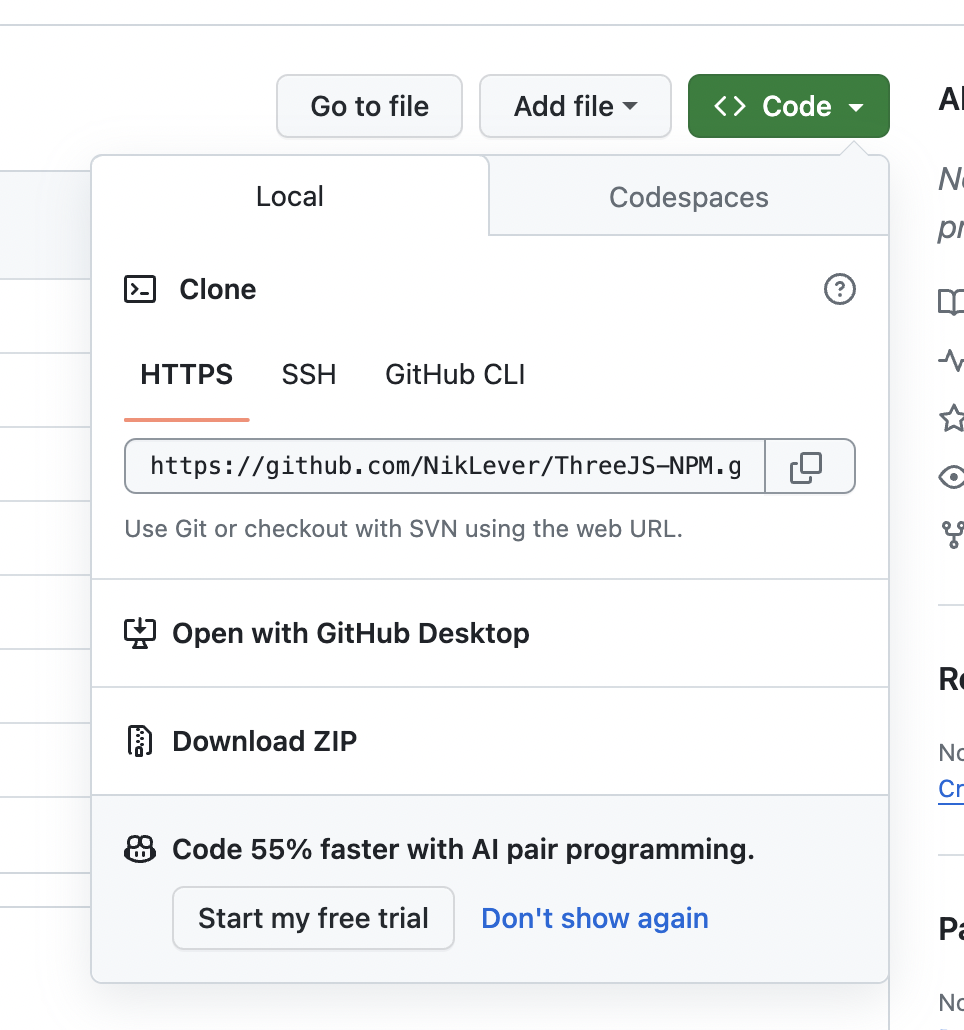
Just click the green Code button and choose Download ZIP. Unzip to a folder of your choice and open the folder in VSCode. To install the dependencies enter
npm install
The package.json file is scanned for dependencies and the node_modules folder is populated with all the packages needed. Recall the scripts we added to package.json. Use
npm run dev
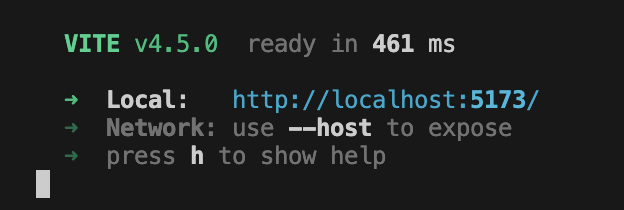
ctrl+click (PC) or cmd+click (Mac) the localhost link to launch the dev server in your browser.
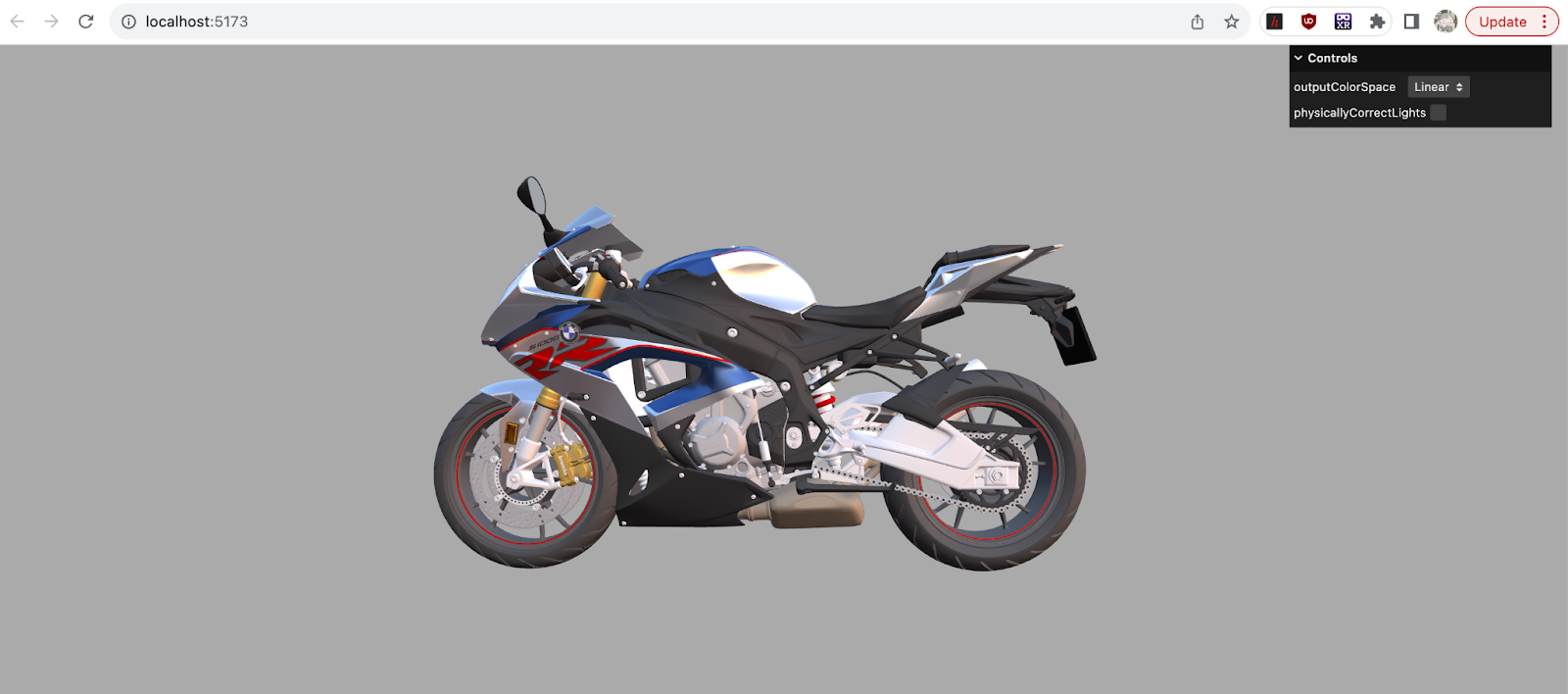
Just a simple example of a Three.JS app created using vite as a build tool.
Take a look at src/index.html. Notice the script. Notice we can import the core Three.JS library from three.
<script type="module">
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
import { GUI } from "three/addons/libs/lil-gui.module.min.js";
import { GLTFLoader } from 'three/addons/loaders/GLTFLoader.js';
import { DRACOLoader } from 'three/addons/loaders/DRACOLoader.js';
import { RGBELoader } from 'three/addons/loaders/RGBELoader.js';
three will be converted into node_modules/three/build/three.module.js and three/addon becomes node_modules/three/examples/jsm. Why? Take a look at package.json in the three folder.
"exports": {
".": {
"import": "./build/three.module.js",
"require": "./build/three.cjs"
},
"./examples/fonts/*": "./examples/fonts/*",
"./examples/jsm/*": "./examples/jsm/*",
"./addons/*": "./examples/jsm/*",
"./src/*": "./src/*",
"./nodes": "./examples/jsm/nodes/Nodes.js"
},
Notice exports. The default export for three, “.”, when used as an import is ./build/three.module.js. Used as a require, something used when creating a nodejs app, uses the classic javascript version ./build.three.cjs. ./addons/* becomes ./examples/jsm/* .
Back to the index.html file. Find the loadGLTF function, line 93.
function loadGLTF(){
const loader = new GLTFLoader( );
const dracoLoader = new DRACOLoader();
dracoLoader.setDecoderPath( 'draco-gltf/' );
loader.setDRACOLoader( dracoLoader );
// Load a glTF resource
loader.load(
// resource URL
'motorcycle.glb',
Notice setDecoderPath is draco-gltf. Since this is not an import, for vite to find it correctly it must be in the public folder.
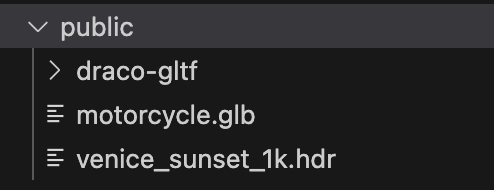
It is simply copied from node_modules/three/examples/jsm/libs/draco/gltf. You can see this folder also contains the glb loaded, motorcycle.glb, and the environment map, venice_sunset_1k.hdr.
For the last step enter
npm run build
Notice a new folder is created, build.
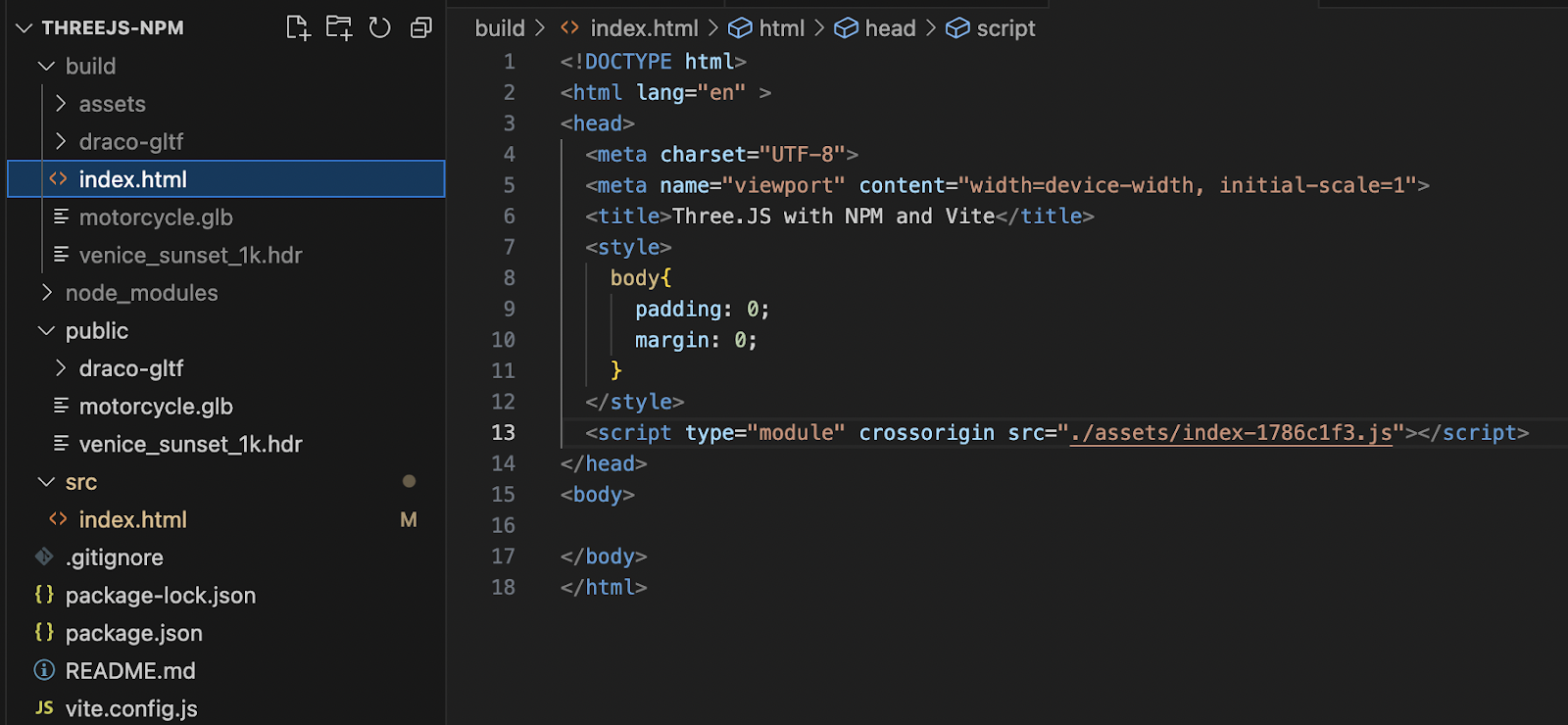
A new index.html is created loading the js file in the assets folder. You might find you need to add a dot before the forward slash.
src=”/assets/index…”
Becomes
src=”./assets/index…”
The contents of the public folder are copied to the build folder. The main script in the assets folder is bundled and minified. The single script now contains the Three.JS library and all the other imports in the index.html file in the src folder.
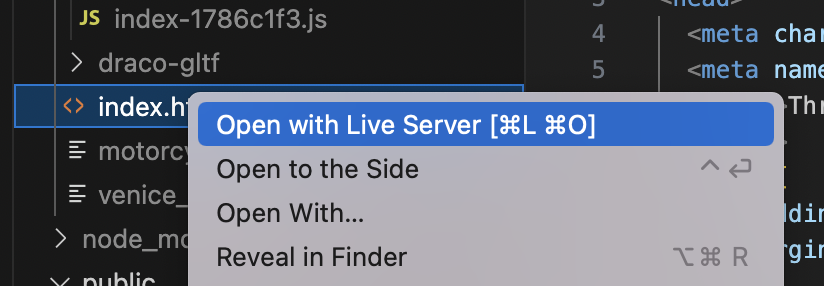
If you have Live Server installed then you can run the app by right clicking on build/index.html and choosing Open with Live Server.
Using npm and vite is a great way to create your Three.JS apps. I hope this short article helps you get started.